When working with WordPress, you may often need to check if a specific plugin is active before executing certain functionality. This is crucial for maintaining compatibility between different plugins and avoiding errors due to missing dependencies. The is_plugin_active
function provides a reliable way to determine whether a plugin is currently active on a WordPress site.
What Is the is_plugin_active
Function?
The is_plugin_active
function is a built-in WordPress function used to verify whether a particular plugin is active. It helps developers ensure that the required plugins are available before executing specific code.
This function is defined in the wp-admin/includes/plugin.php
file and is primarily used in the admin area. However, it can still be utilized in front-end code as long as the necessary file is included.
Function Syntax
is_plugin_active( $plugin )
Parameter: $plugin
– This is the plugin’s file path relative to the wp-content/plugins/
directory. The typical format is:
'plugin-folder/plugin-file.php'
How to Use is_plugin_active
in WordPress
Below are different ways to use the is_plugin_active
function in various scenarios.
1. Checking If a Plugin Is Active
If you need to determine whether a plugin is active before executing some code, use the following approach:
if ( is_plugin_active('woocommerce/woocommerce.php') ) {
// Execute code if WooCommerce is active
echo 'WooCommerce is installed and active!';
}
In the example above, the function checks if WooCommerce is active before executing the associated code.
2. Using is_plugin_active
in Non-Admin Pages
Since is_plugin_active
is defined in an admin-specific file, it may not always work outside the admin environment. To use it on the front-end, you need to include the necessary file:
include_once( ABSPATH . 'wp-admin/includes/plugin.php' );
if ( is_plugin_active('elementor/elementor.php') ) {
// Actions to take if Elementor is active
}
This ensures the function is available before calling it.
3. Checking for a Plugin in a Theme or Plugin
If you are developing a theme or plugin that integrates with another plugin, checking whether it is active can prevent errors and improve compatibility:
function check_required_plugins() {
include_once( ABSPATH . 'wp-admin/includes/plugin.php' );
if ( !is_plugin_active('contact-form-7/wp-contact-form-7.php') ) {
echo 'Warning: Contact Form 7 is not installed or activated.';
}
}
add_action( 'admin_notices', 'check_required_plugins' );
Here, the function checks whether Contact Form 7 is active. If not, it displays an admin notice.
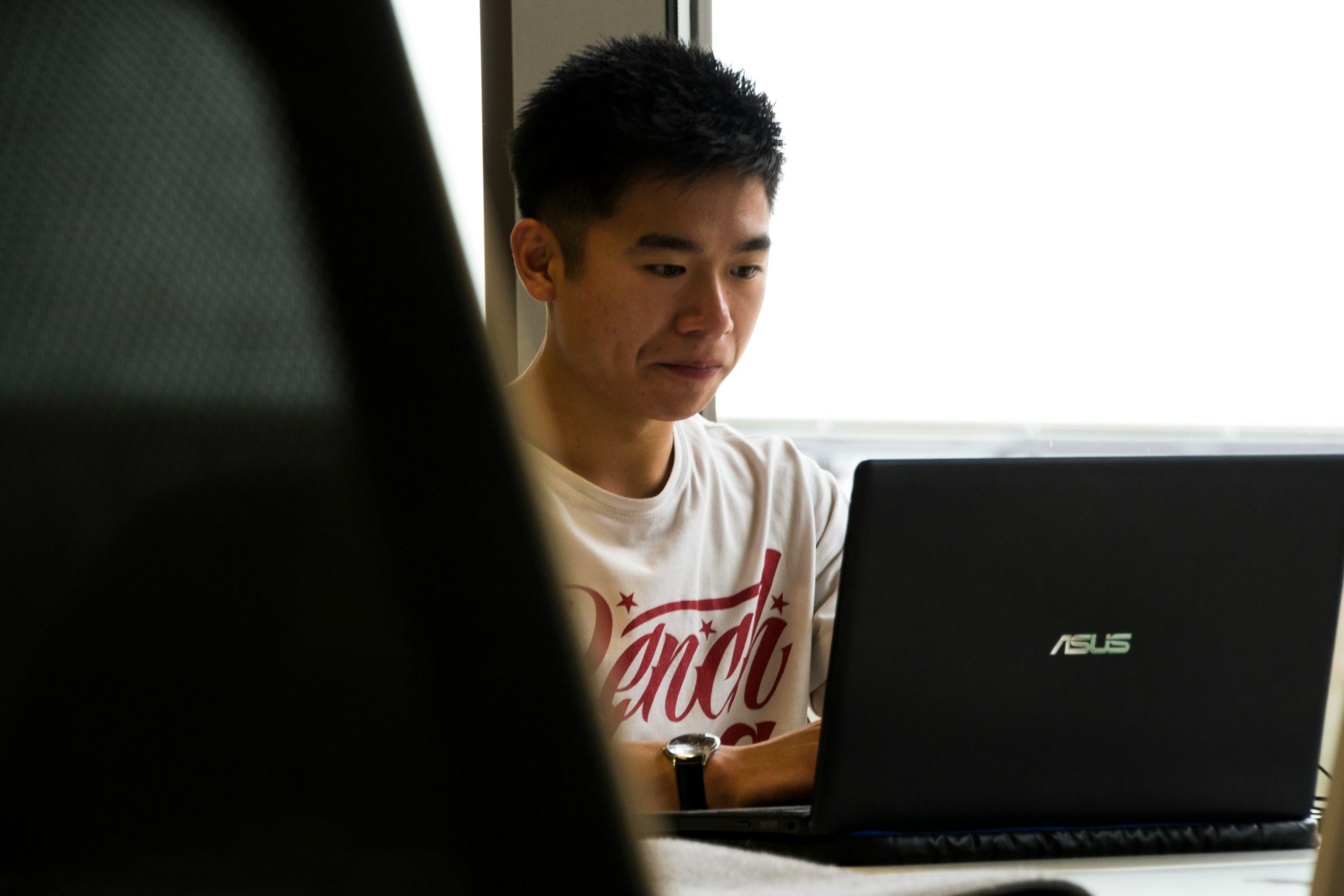
Alternative Approaches
While is_plugin_active
is a useful function, there are alternative methods to check for active plugins:
1. Using get_option
WordPress stores active plugins in the active_plugins
option within the database. You can retrieve this data directly:
$active_plugins = get_option('active_plugins');
if ( in_array('classic-editor/classic-editor.php', $active_plugins) ) {
echo 'Classic Editor is active!';
}
2. Checking for a Plugin’s Defined Function or Class
If a plugin provides specific functions or classes, you can verify its presence using function_exists
or class_exists
:
if ( function_exists('woocommerce') ) {
echo 'WooCommerce is active!';
}
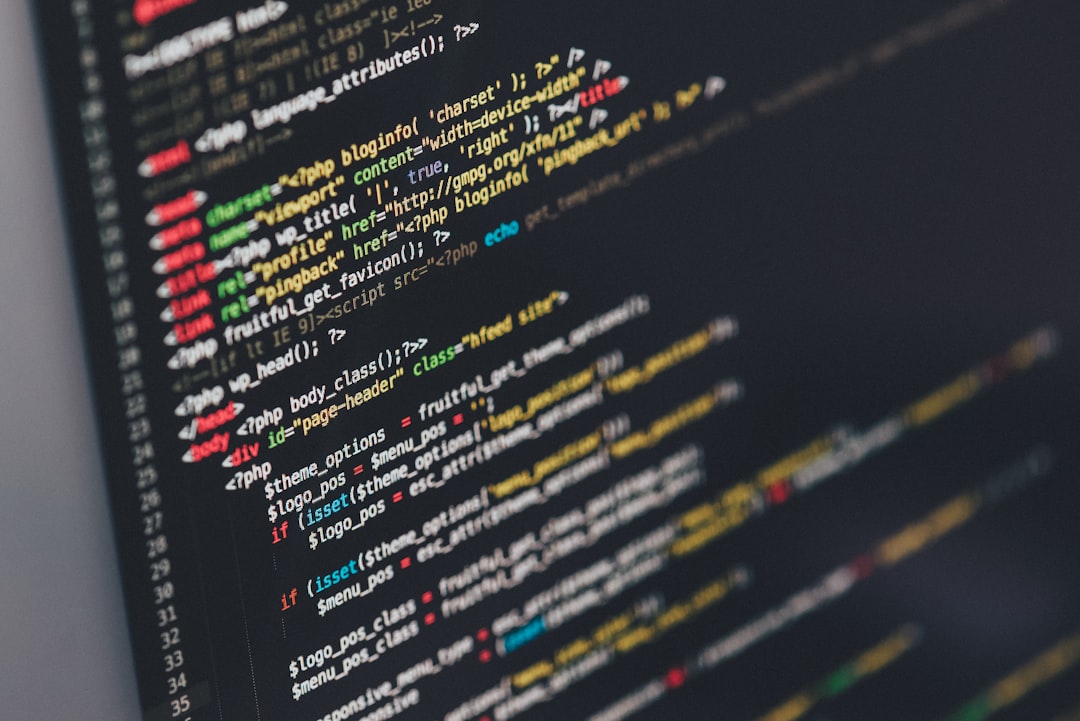
Common Issues and Troubleshooting
While using is_plugin_active
, you may encounter some common issues:
- Function not found: Ensure you include
wp-admin/includes/plugin.php
if using the function outside the admin area. - Incorrect file path: The function requires the exact plugin file path. An incorrect path will return a false negative.
- Caching problems: Sometimes, WordPress caching might prevent changes in plugin status from reflecting immediately.
Conclusion
The is_plugin_active
function is a powerful and reliable way to check plugin activation status in WordPress. It allows developers to ensure that required plugins are installed before running dependent code, preventing compatibility issues and improving user experience.
By understanding how to use this function properly, developers can build more robust integrations and avoid common pitfalls. Whether you are checking for plugin dependencies in a theme, plugin, or admin notice, is_plugin_active
is a valuable tool in WordPress development.