In the world of programming and software development, automation is crucial. One common task when working with APIs and web services is passing parameters into a URL for requests. Python’s Requests library offers a simple and efficient way to achieve this, making it a valuable tool for developers working with HTTP protocols. Whether you’re retrieving data from a REST API or sending information to a server, understanding how to pass parameters into a URL is essential.
What Are URL Parameters?
URL parameters, also known as query strings, are key-value pairs appended to the URL to provide additional context or instruction for your request. They typically follow the format:
?key1=value1&key2=value2
For example, a weather API might use parameters like city
and unit
to specify the city for which weather data is requested and whether you prefer the temperature in Celsius or Fahrenheit.
With Python and its Requests
library, you can easily pass parameters into a URL to customize your HTTP requests and retrieve the desired response.
Using Python’s Requests Library
The Requests
library makes it simple to add parameters to a URL when making GET requests. Let’s explore the process step by step with examples.
Step 1: Installing the Requests Library
If you don’t already have the library installed, you can quickly install it using pip
:
pip install requests
Step 2: Basic GET Request with Parameters
To include parameters in a GET request, use the params
argument of the requests.get()
method. Here’s an example:
import requests # Define the URL and parameters url = "https://api.example.com/data" params = { "key1": "value1", "key2": "value2" } # Make the GET request response = requests.get(url, params=params) # Print the Response print(response.url) print(response.text)
In the example above, we define a dictionary (params
) containing the parameters and their values. The library automatically encodes the parameters into the URL when the request is made.
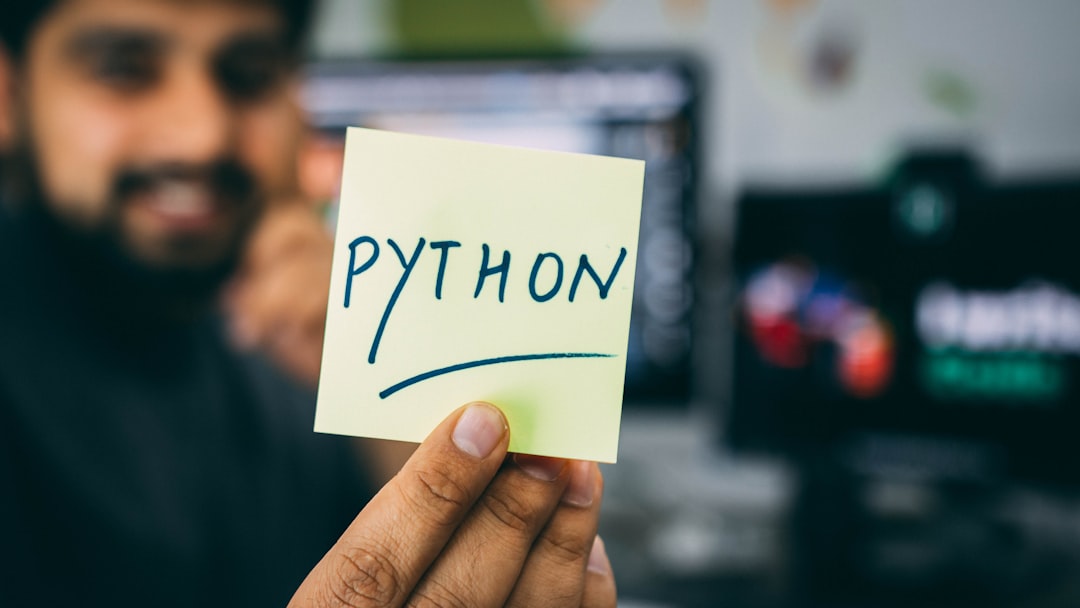
When you print the URL as shown above, you’ll notice the parameters are appended to it in the proper query string format. This feature allows you to pass any number of key-value pairs while keeping your code clean and organized.
Step 3: Advanced Parameter Management
In more complex scenarios, you might need to handle dynamic or nested parameters. The Requests
library can accommodate these as well:
- Dynamic Parameters: If you build URLs dynamically based on user input or other conditions, you can generate the parameter dictionary programmatically.
- Nested Parameters: Some APIs allow nested structures (e.g., JSON objects) to be passed as URL parameters by encoding them appropriately. In such cases, libraries like
urllib.parse
can assist with formatting.
Example: Dynamic Parameter Creation
import requests url = "https://api.example.com/search" search_criteria = ["python", "requests", "parameters"] # Create dynamic parameters params = {"query": " ".join(search_criteria)} response = requests.get(url, params=params) print(response.url)
In this scenario, we dynamically create a search query using a list and pass it as a parameter to the URL. The resulting URL will look like: https://api.example.com/search?query=python+requests+parameters
.
Benefits of Using the Requests Library
Passing parameters into URLs with the Requests
library offers several advantages:
- Simplicity: The syntax for handling parameters is straightforward, reducing the learning curve for new developers.
- Efficiency: Automated encoding of parameters ensures your data is correctly formatted for the server.
- Flexibility: It supports advanced use cases like dynamic and nested parameters, making it suitable for highly customized requests.
When to Use URL Parameters
URL parameters are generally used for read operations since their data is visible in the browser or API logs. For example:
- Filtering database records through an API (e.g., fetching data within a date range).
- Customizing API responses by specifying return fields or formats.
- Paginating data, using parameters like
page
andlimit
.
Tips for Avoiding Common Pitfalls
While URL parameters are simple to use, keep the following points in mind:
- Parameter Length Limitations: Most web servers impose limits on URL length. If your parameters are too long (e.g., a complex JSON structure), consider using a POST request.
- Proper Encoding: Special characters in parameter values (e.g., spaces) must be URL-encoded to avoid issues.
- Security Concerns: Avoid including sensitive data in URL parameters, as they can be logged or cached by servers and browsers.
Conclusion
Passing parameters into a URL for Python Requests is a crucial skill for developers working with APIs and web services. The Requests
library simplifies this task, offering an intuitive and flexible way to handle parameters in your requests. By mastering these techniques, you’ll be better equipped to retrieve data efficiently and customize your interactions with web services.
With the tips and examples provided here, you can begin confidently using URL parameters in your Python projects.