Building dynamic web applications often requires integrating third-party services for seamless functionalities. One common integration is adding Stripe—a popular payment processing platform—to a Dash app. Dash, a web-based Python framework for building interactive data visualization interfaces, is not inherently designed for payment processing, but with the right approach, you can effectively integrate Stripe to power payments in your app.
In this article, we’ll explore whether you can add Stripe to a Dash app, how it works, and a step-by-step guide to implementing it. Let’s break it down!
What Is Stripe?
Stripe is a widely-used platform that allows developers to accept payments, manage subscriptions, and handle transactions online. Its robust API and developer-friendly environment make it a top choice for integrating payment gateways in various types of web applications.
By combining Stripe with Dash, you can enable payments, monetize your app, or even incorporate donation systems. However, there’s a catch: while Dash excels in creating sophisticated user interfaces with pure Python, adding a payment gateway may require some knowledge of JavaScript, HTML, and backend development.
Can You Add Stripe to a Dash App?
The short answer is yes, you can add Stripe to a Dash app! But it requires a workaround since Dash doesn’t natively support Stripe or payment processing. The integration process typically includes two key steps:
- Setting up a Stripe account and configuring your payment methods through their dashboard and API keys.
- Embedding a payment flow within your Dash app. This can require basic web development techniques involving JavaScript and HTML, communicated seamlessly with Dash’s Python callbacks.
It may seem a bit tricky at first, but with some guiding principles and examples, you can successfully accomplish this task even without being an expert in web development technologies outside of Python!
How to Add Stripe to a Dash App: Step-by-Step
Here’s a simplified approach to integrating Stripe with your Dash app:
1. Setting Up Your Stripe Account
Before doing anything in your Dash app, create a Stripe account at their official website. Once registered, navigate to the Stripe Dashboard where you’ll find your test API keys. These keys will allow you to simulate payments and test the integration process.
Make sure to switch to live keys when deploying your app in production mode.
2. Create the Payment Flow
Stripe offers Checkout, a pre-built hosted payment page that can be easily embedded into your web applications. This requires a small amount of JavaScript to trigger the Stripe Checkout page. Alternatively, you can build a custom payment form using the Stripe Elements library.
To integrate in Dash, you’ll typically need to:
- Embed the JavaScript component for the Stripe checkout form in your app’s layout.
- Use Dash’s `html.Div` or `html.Script` components to include the Stripe functionality.
- Include server-side logic (backend Python code) to validate and process the payments.
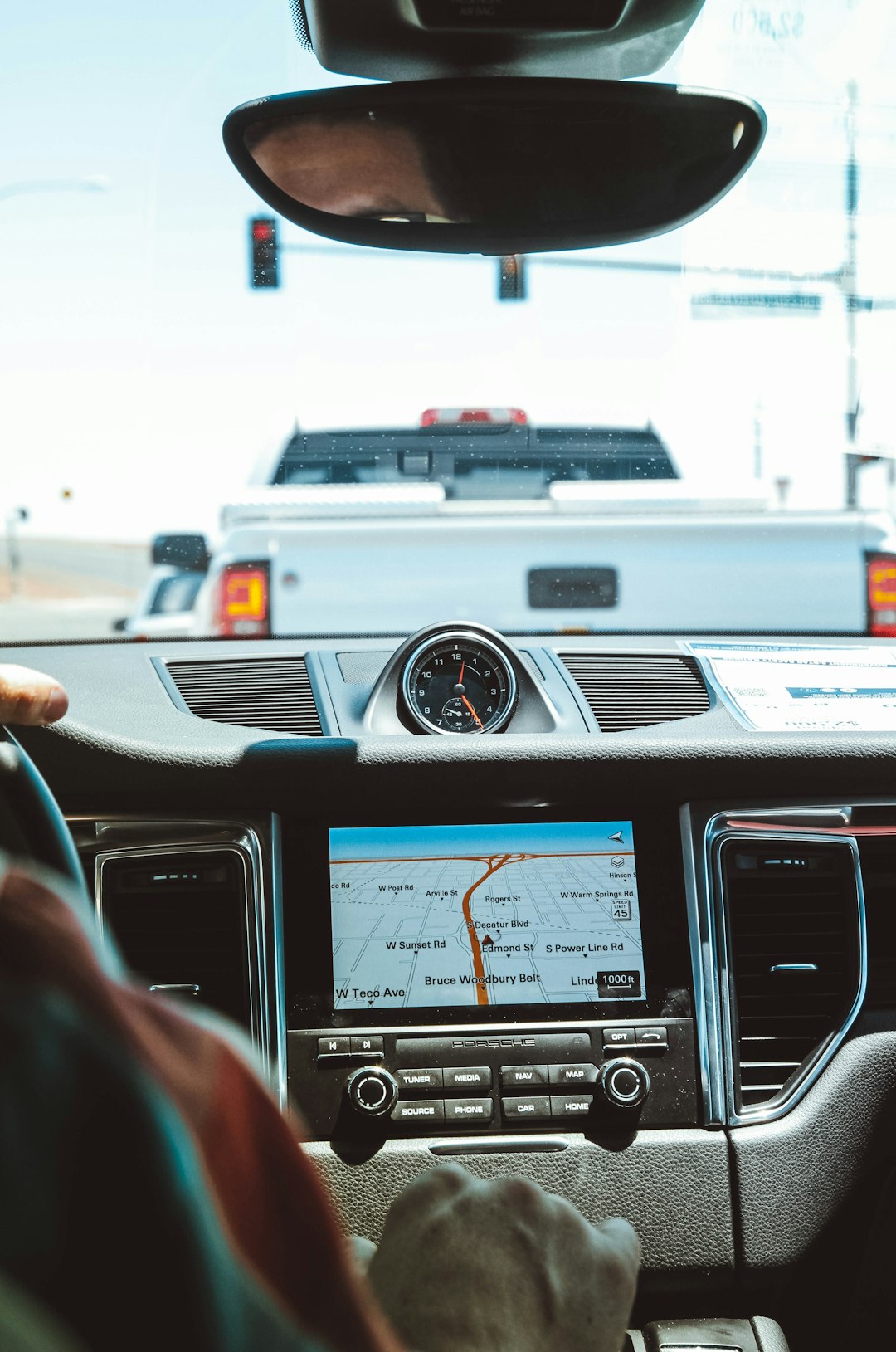
Here’s an example of a simple Stripe payment button. Place this code in your Dash layout:
``` html.Div([ html.Button("Pay Now", id="pay-button"), html.Script(src="https://js.stripe.com/v3/"), html.Script(""" document.getElementById('pay-button').addEventListener('click', function() { var stripe = Stripe('your-publishable-key'); stripe.redirectToCheckout({ sessionId: 'your-session-id' }); }); """) ]) ```
In the above code, replace ‘your-publishable-key’ and ‘your-session-id’ with your actual Stripe keys.
3. Implementing Python Callbacks
Next, use Dash’s callback functions to dynamically generate session IDs or handle responses when users interact with your app. You may need to set up a Flask microservice or use Dash’s built-in server to handle the backend logic.
For example, use `@app.callback` to trigger events when a user clicks the “Pay Now” button.
4. Testing the Integration
Stripe provides an extensive testing mode where you can use special dummy card numbers to simulate various payment scenarios. This ensures your implementation is secure and error-free before moving to production.
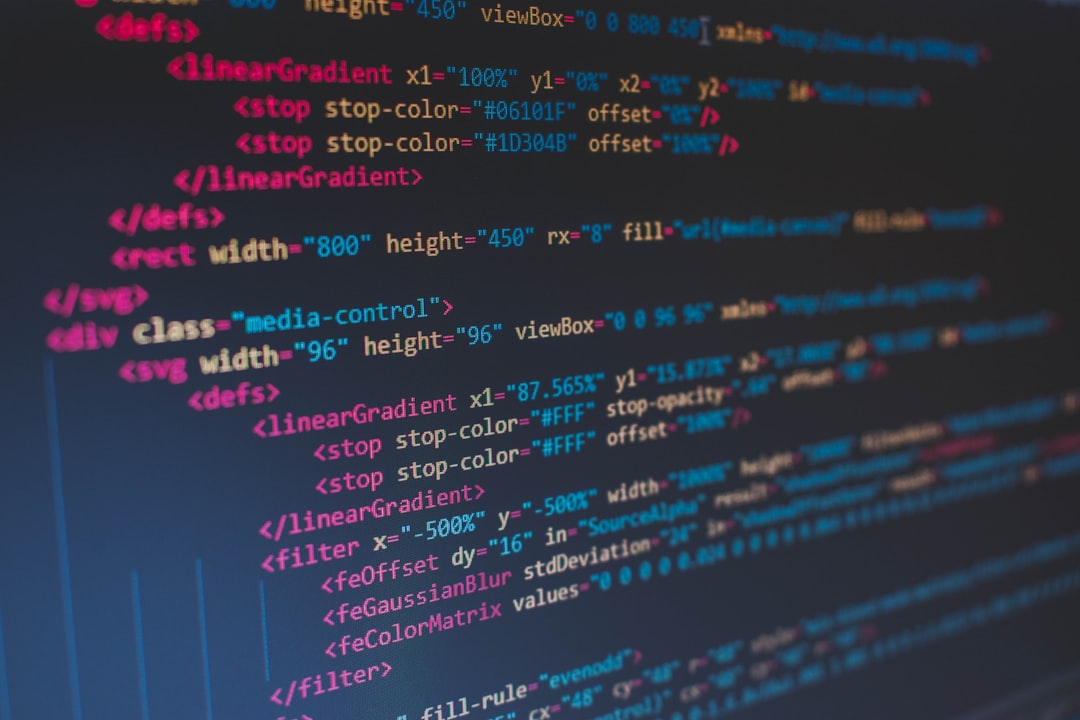
Best Practices for Adding Stripe to Dash
- Use Environment Variables: Store sensitive Stripe keys as environment variables to keep them secure.
- Validate User Input: Check all user inputs in payment forms to prevent fraud or errors.
- Follow Security Guidelines: Stripe mandates PCI compliance, so ensure your app aligns with security best practices.
Stripe also provides rich analytic tools to track your transactions and payment activity, giving you deeper insight into your app’s financial performance once it integrates with Dash.
Conclusion
Adding Stripe to a Dash app opens up exciting possibilities for building applications that require payment functionality. While it does involve some extra steps, like working with JavaScript and backend logic, the effort pays off if you’re looking to monetize your web app or add donations.
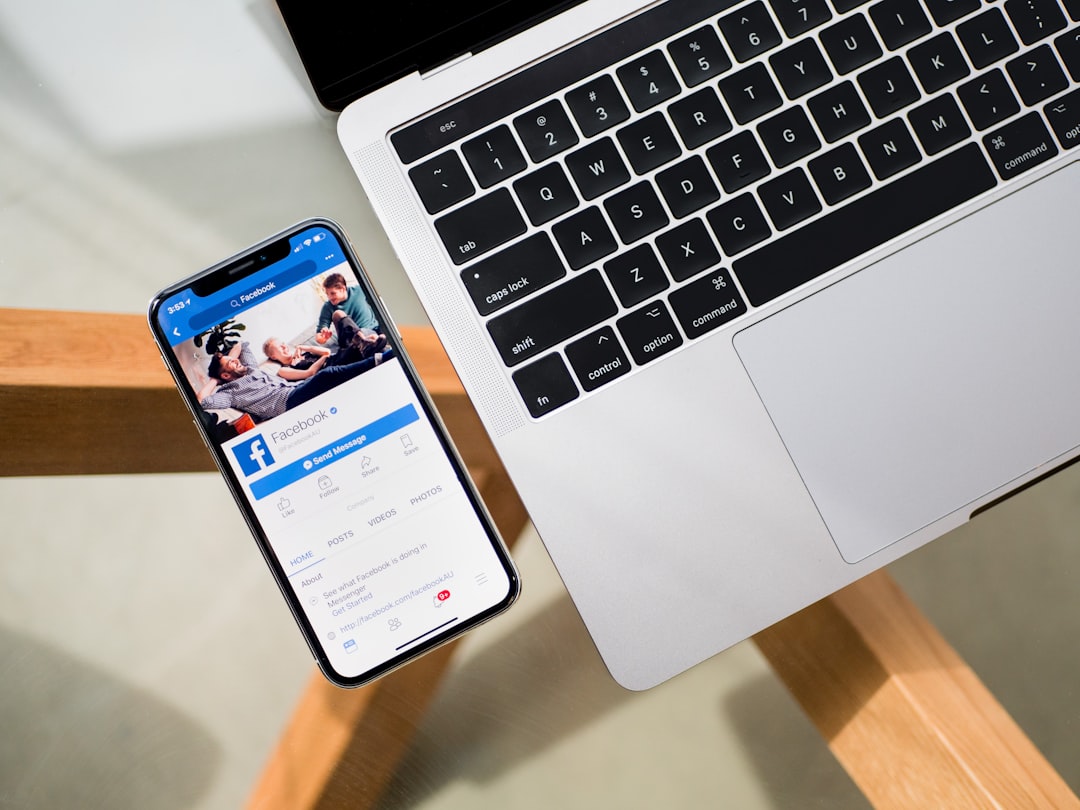
With careful implementation and testing, you can seamlessly combine the two platforms to create a robust, revenue-generating application. Whether you’re building a subscription service, selling products, or enabling donations, Stripe and Dash together provide a powerful combination.